Import numpy as np import matplotlib.pyplot as plt from PIL import Image
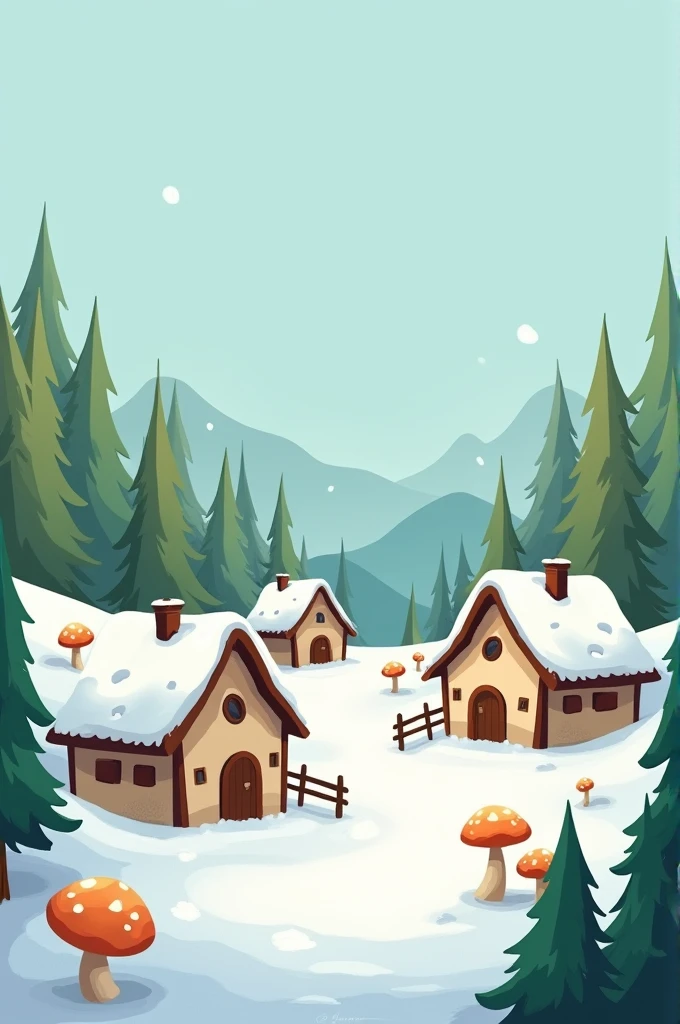

import numpy as np import matplotlib.pyplot as plt from PIL import Image, ImageDraw, ImageFont # Set up the canvas size width, height = 800, 600 image = Image.new('RGB', (width, height), (135, 206, 250)) # Sky blue background draw = ImageDraw.Draw(image) # Define the colors roof_color = (139, 69, 19) # Brown wall_color = (255, 228, 196) # Beige window_color = (0, 0, 0) # Black door_color = (139, 69, 19) # Brown snow_color = (255, 250, 250) # White tree_color = (34, 139, 34) # Forest green mushroom_color = (255, 140, 0) # Orange cloud_color = (255, 255, 255) # White # Define the house dimensions house_width = 200 house_height = 150 roof_height = 70 # Define the landscape landscape_height = 400 tree_width = 50 tree_height = 100 tree_count = 20 mushroom_count = 10 # Draw the landscape draw.rectangle((0, height - landscape_height, width, height), fill=(34, 139, 34)) # Grass # Draw the snow draw.rectangle((0, height - landscape_height, width, height), fill=snow_color) # Draw the trees for i in range(tree_count): tree_x = np.random.randint(tree_width, width - tree_width) tree_y = height - landscape_height + np.random.randint(0, landscape_height - tree_height) draw.rectangle((tree_x, tree_y, tree_x + tree_width, tree_y + tree_height), fill=tree_color) # Draw the mushrooms for i in range(mushroom_count): mushroom_x = np.random.randint(tree_width, width - tree_width) mushroom_y = height - landscape_height + np.random.randint(0, landscape_height - 50) draw.ellipse((mushroom_x - 20, mushroom_y, mushroom_x + 20, mushroom_y + 40), fill=mushroom_color) draw.ellipse((mushroom_x - 10, mushroom_y + 20, mushroom_x + 10, mushroom_y + 40), fill=(255, 255, 255)) # Draw the houses house_spacing = 100 for i in range(3): house_x = i * (house_width + house_spacing) house_y = height - landscape_height - house_height draw.rectangle((house_x, house_y, house_x + house_width, house_y + house_height), fill=wall_color) # Draw the roof
Prompts
Prompts kopieren
import numpy as np
import matplotlib.pyplot as plt
from PIL import Image, ImageDraw, ImageFont
# Set up the canvas size
width, height = 800, 600
image = Image.new('RGB', (width, height), (135, 206, 250)) # Sky blue background
draw = ImageDraw.Draw(image)
# Define the colors
roof_color = (139, 69, 19) # Brown
wall_color = (255, 228, 196) # Beige
window_color = (0, 0, 0) # Black
door_color = (139, 69, 19) # Brown
snow_color = (255, 250, 250) # White
tree_color = (34, 139, 34) # Forest green
mushroom_color = (255, 140, 0) # Orange
cloud_color = (255, 255, 255) # White
# Define the house dimensions
house_width = 200
house_height = 150
roof_height = 70
# Define the landscape
landscape_height = 400
tree_width = 50
tree_height = 100
tree_count = 20
mushroom_count = 10
# Draw the landscape
draw.rectangle((0, height - landscape_height, width, height), fill=(34, 139, 34)) # Grass
# Draw the snow
draw.rectangle((0, height - landscape_height, width, height), fill=snow_color)
# Draw the trees
for i in range(tree_count):
tree_x = np.random.randint(tree_width, width - tree_width)
tree_y = height - landscape_height + np.random.randint(0, landscape_height - tree_height)
draw.rectangle((tree_x, tree_y, tree_x + tree_width, tree_y + tree_height), fill=tree_color)
# Draw the mushrooms
for i in range(mushroom_count):
mushroom_x = np.random.randint(tree_width, width - tree_width)
mushroom_y = height - landscape_height + np.random.randint(0, landscape_height - 50)
draw.ellipse((mushroom_x - 20, mushroom_y, mushroom_x + 20, mushroom_y + 40), fill=mushroom_color)
draw.ellipse((mushroom_x - 10, mushroom_y + 20, mushroom_x + 10, mushroom_y + 40), fill=(255, 255, 255))
# Draw the houses
house_spacing = 100
for i in range(3):
house_x = i * (house_width + house_spacing)
house_y = height - landscape_height - house_height
draw.rectangle((house_x, house_y, house_x + house_width, house_y + house_height), fill=wall_color)
# Draw the roof
Info
Checkpoint & LoRA
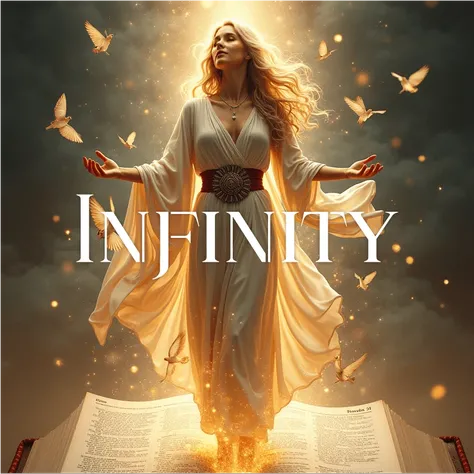
Checkpoint
SeaArt Infinity
#Landschaft
#Zeichentrick
#Szenografie
#SeaArt Infinity
0 Kommentar(e)
0
0
0