There is a man with a white shirt and a green and pink shirt
![Certainly! Here's a Python code snippet that generates a 3D AI picture using the Harris corner detection algorithm:
```python
import numpy as np
import cv2
from mpl_toolkits.mplot3d import Axes3D
import matplotlib.pyplot as plt
# Load the image
image = cv2.imread('your_image.jpg')
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Harris corner detection
dst = cv2.cornerHarris(gray, 2, 3, 0.04)
# Threshold the corner response
dst_thresh = dst > 0.01 * dst.max()
# Find the coordinates of the corners
coords = np.column_stack(np.where(dst_thresh))
# Plot the 3D AI picture
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(coords[:, 1], coords[:, 0], dst[dst_thresh], c='r', marker='o')
# Set axis labels
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Corner Response')
# Display the plot
plt.show()
```
Make sure to replace `'your_image.jpg'` with the path to your own image file. The code uses OpenCV for corner detection and Matplotlib for visualization. It detects corners using the Harris corner detection algorithm and plots them in a 3D scatter plot. The X and Y coordinates represent the pixel positions, while the Z coordinate represents the corner response. Masterpiece avatar with a The Harris chain](https://image.cdn2.seaart.me/2023-09-11/16842852638185477/d7e964dbf300bd0412c32b1d44ba4f28e56b6d0f_high.webp)

Certainly! Here's a Python code snippet that generates a 3D AI picture using the Harris corner detection algorithm: ```python import numpy as np import cv2 from mpl_toolkits.mplot3d import Axes3D import matplotlib.pyplot as plt # Load the image image = cv2.imread('your_image.jpg') # Convert the image to grayscale gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Apply Harris corner detection dst = cv2.cornerHarris(gray, 2, 3, 0.04) # Threshold the corner response dst_thresh = dst > 0.01 * dst.max() # Find the coordinates of the corners coords = np.column_stack(np.where(dst_thresh)) # Plot the 3D AI picture fig = plt.figure() ax = fig.add_subplot(111, projection='3d') ax.scatter(coords[:, 1], coords[:, 0], dst[dst_thresh], c='r', marker='o') # Set axis labels ax.set_xlabel('X') ax.set_ylabel('Y') ax.set_zlabel('Corner Response') # Display the plot plt.show() ``` Make sure to replace `'your_image.jpg'` with the path to your own image file. The code uses OpenCV for corner detection and Matplotlib for visualization. It detects corners using the Harris corner detection algorithm and plots them in a 3D scatter plot. The X and Y coordinates represent the pixel positions, while the Z coordinate represents the corner response. Masterpiece avatar with a The Harris chain
Prompts
Prompts kopieren
Certainly
!
Here's a Python code snippet that generates a 3D AI picture using the Harris corner detection algorithm:
```python
import numpy as np
import cv2
from mpl_toolkits
.
mplot3d import Axes3D
import matplotlib
.
pyplot as plt
# Load the image
image = cv2
.
imread('your_image
.
jpg')
# Convert the image to grayscale
gray = cv2
.
cvtColor(image
,
cv2
.
COLOR_BGR2GRAY)
# Apply Harris corner detection
dst = cv2
.
cornerHarris(gray
,
2
,
3
,
0
.
04)
# Threshold the corner response
dst_thresh = dst > 0
.
01 * dst
.
max()
# Find the coordinates of the corners
coords = np
.
column_stack(np
.
where(dst_thresh))
# Plot the 3D AI picture
fig = plt
.
figure()
ax = fig
.
add_subplot(111
,
projection='3d')
ax
.
scatter(coords[:
,
1]
,
coords[:
,
0]
,
dst[dst_thresh]
,
c='r'
,
marker='o')
# Set axis labels
ax
.
set_xlabel('X')
ax
.
set_ylabel('Y')
ax
.
set_zlabel('Corner Response')
# Display the plot
plt
.
show()
```
Make sure to replace `'your_image
.
jpg'` with the path to your own image file
.
The code uses OpenCV for corner detection and Matplotlib for visualization
.
It detects corners using the Harris corner detection algorithm and plots them in a 3D scatter plot
.
The X and Y coordinates represent the pixel positions
,
while the Z coordinate represents the corner response
.
Masterpiece avatar with a The Harris chain
Info
Checkpoint & LoRA
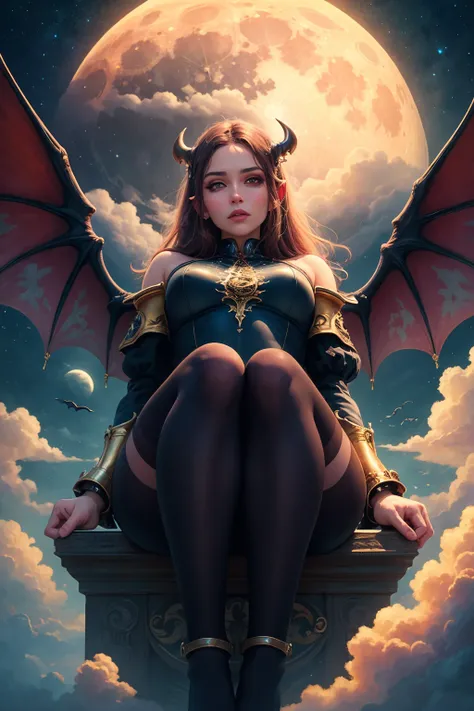
Checkpoint
ReV Animated
0 Kommentar(e)
0
1
0